Getting Started with pplx-api
You can access pplx-api using HTTPS requests. Authenticating involves the following steps:
Start by visiting the Perplexity API Settings page.
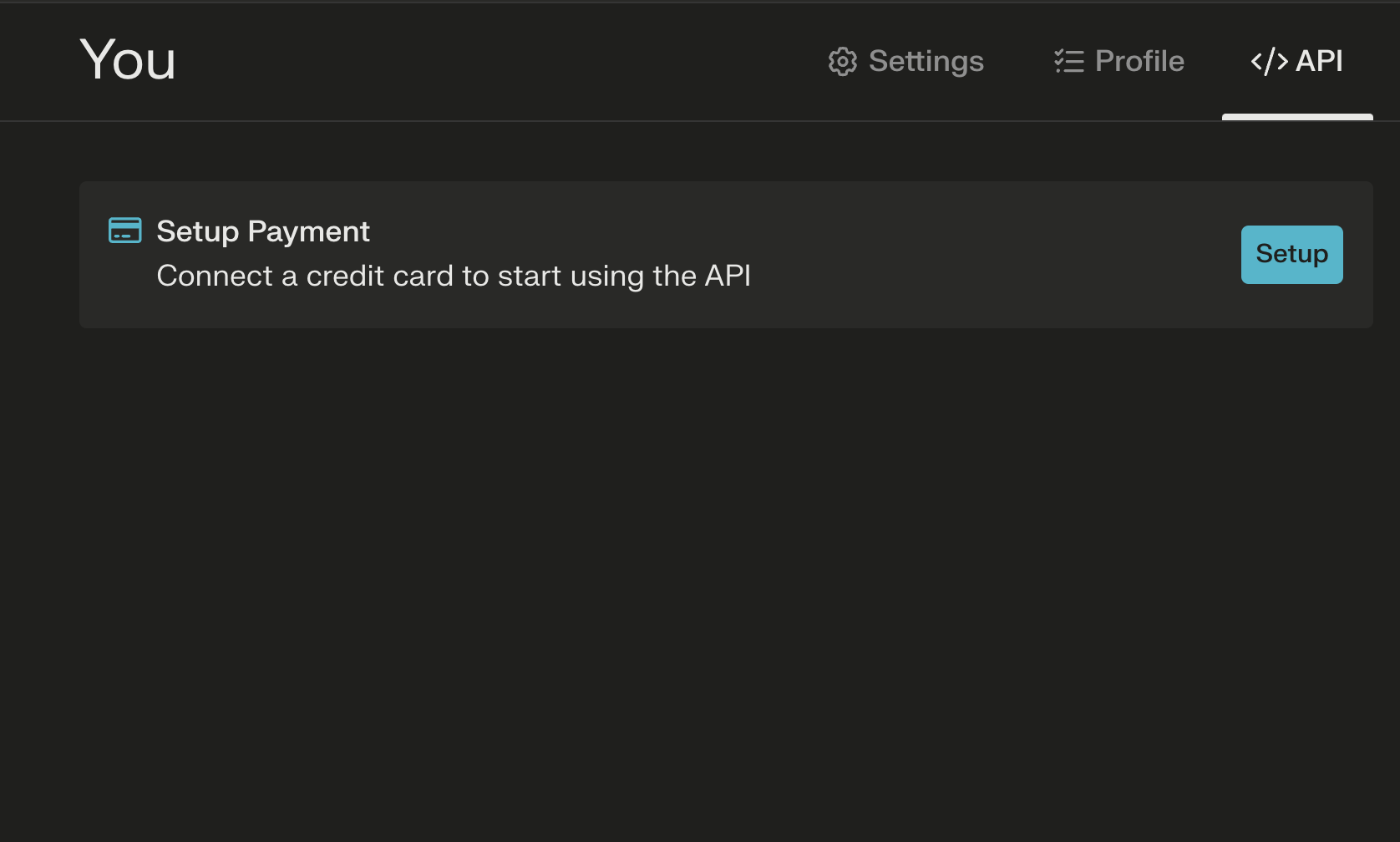
Register your credit card to get started. This step will not charge your credit card. Rather, it stores payment information for later API usage.
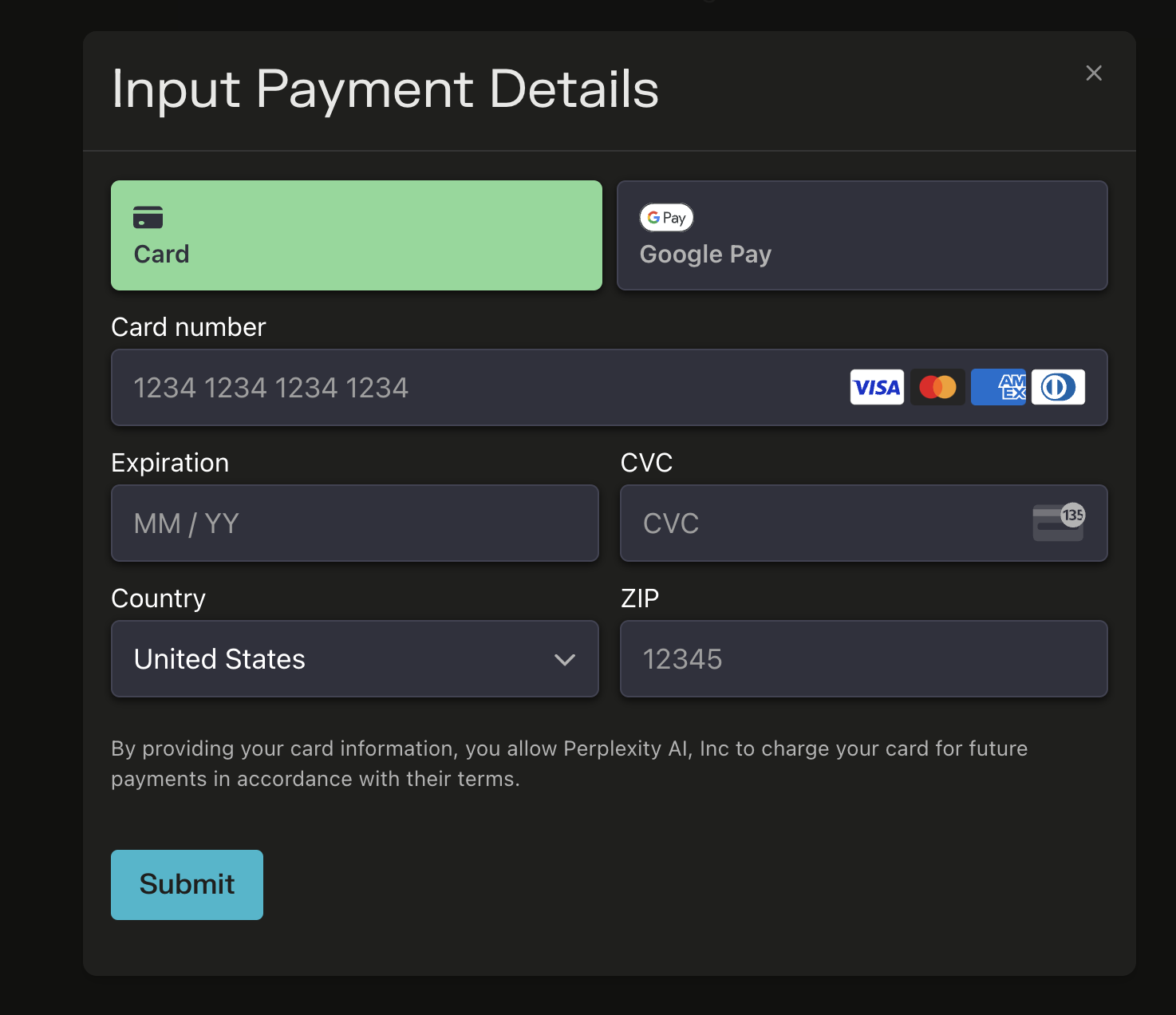
After providing your payment information, you are ready to purchase credits. API keys can only be generated when your balance is nonzero. Note that pro users receive $5 of free credits on the first day of every month. If you recently purchased Pro, please wait up to 10 minutes for your free $5 credit to be visible in your available balance.
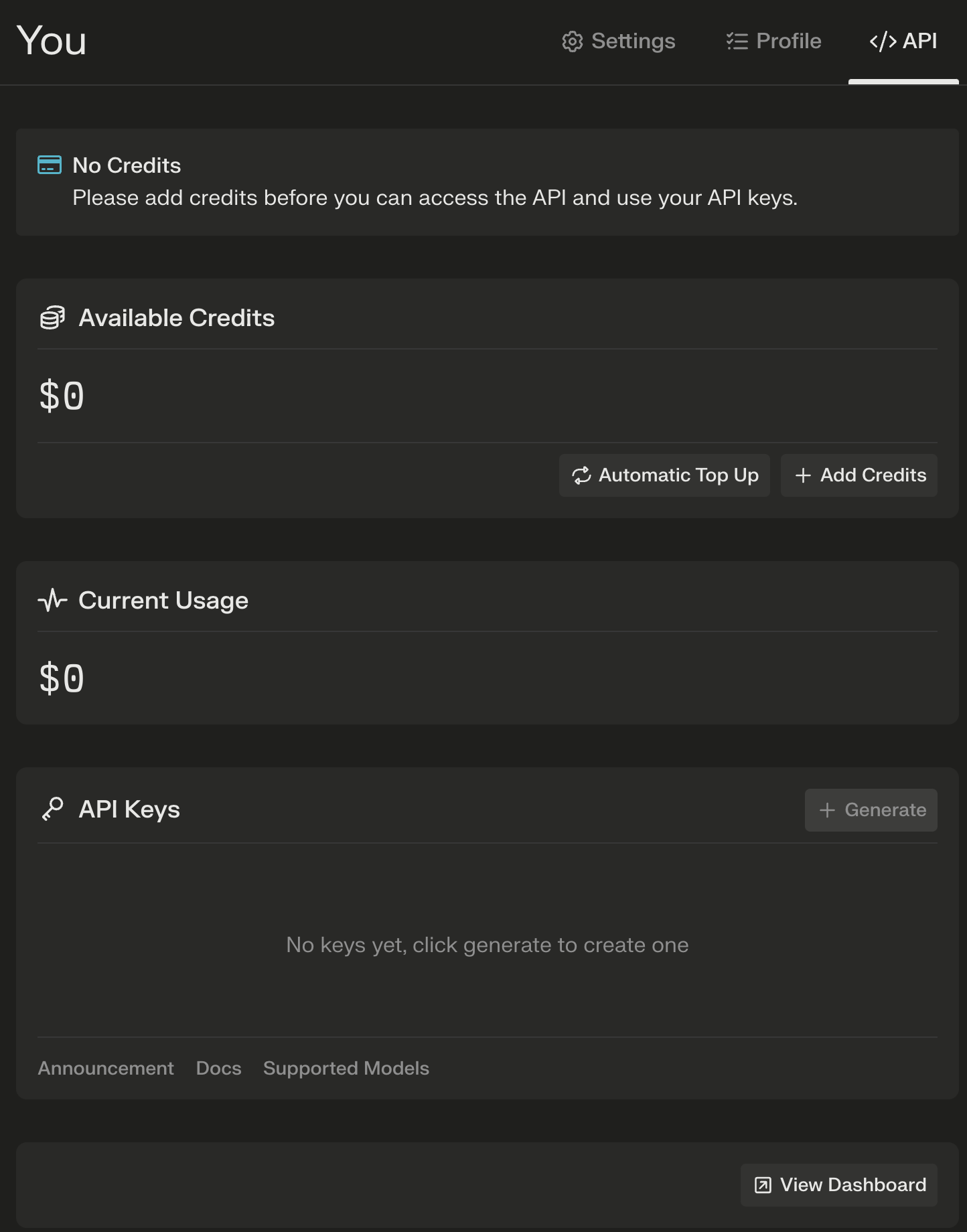
Generate an API key. The API key is a long-lived access token that can be used until it is manually refreshed or deleted.
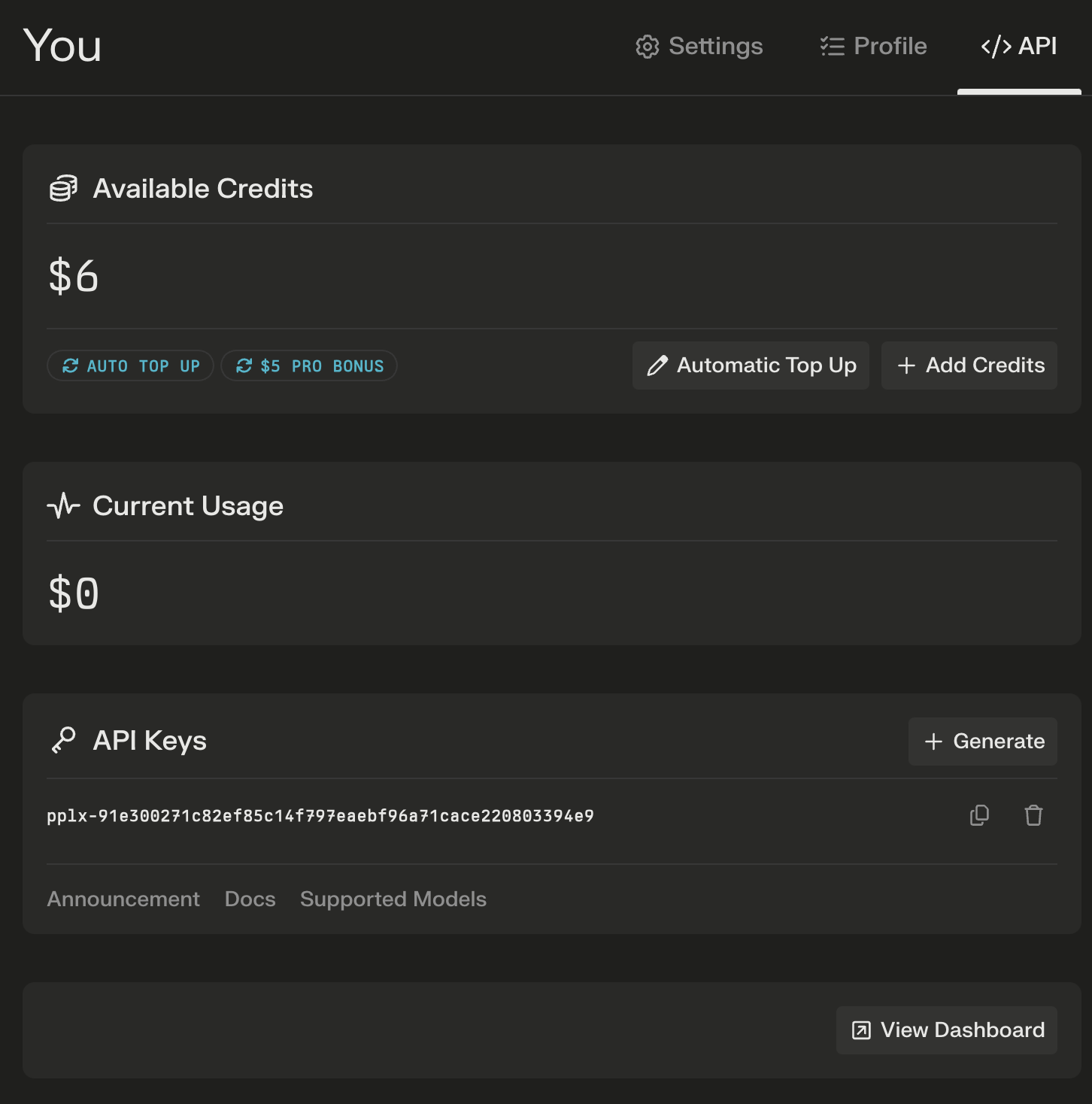
Send the API key as a bearer token in the Authorization header with each pplx-api request.
When you run out of credits, your API keys will be blocked until you add to your credit balance. You can avoid this by configuring "Automatic Top Up", which refreshes your balance whenever you drop below $2.
Our supported models are listed on the "Supported Models" page. The API is conveniently OpenAI client-compatible for easy integration with existing applications.
from openai import OpenAI
YOUR_API_KEY = "INSERT API KEY HERE"
messages = [
{
"role": "system",
"content": (
"You are an artificial intelligence assistant and you need to "
"engage in a helpful, detailed, polite conversation with a user."
),
},
{
"role": "user",
"content": (
"How many stars are in the universe?"
),
},
]
client = OpenAI(api_key=YOUR_API_KEY, base_url="https://api.perplexity.ai")
# chat completion without streaming
response = client.chat.completions.create(
model="mistral-7b-instruct",
messages=messages,
)
print(response)
# chat completion with streaming
response_stream = client.chat.completions.create(
model="mistral-7b-instruct",
messages=messages,
stream=True,
)
for response in response_stream:
print(response)
Updated about 2 months ago